@ -10,6 +10,9 @@ When we think about writing code, we always want to ensure our code is readable.
[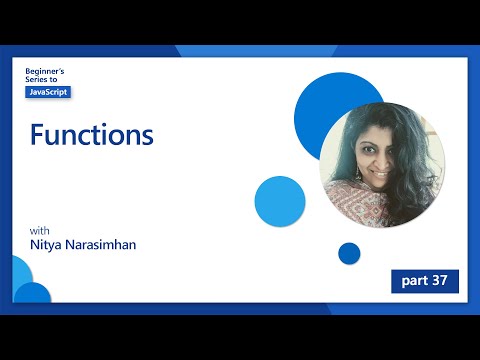](https://youtube.com/watch?v=XgKsD6Zwvlc "Methods and Functions")
> Click the image above for a video about methods and functions.
## Functions
At its core, a function is a block of code we can execute on demand. This is perfect for scenarios where we need to perform the same task multiple times; rather than duplicating the logic in multiple locations (which would make it hard to update when the time comes), we can centralize it in one location, and call it whenever we need the operation performed - you can even call functions from other functions!.
@ -10,6 +10,7 @@ This lesson covers the basics of JavaScript, the language that provides interact
[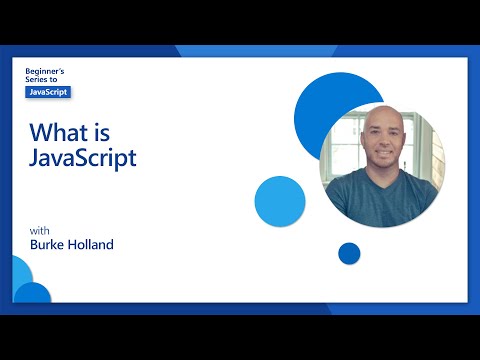](https://youtube.com/watch?v=Q_CRM2lXXBg "Arrays and Loops")
> Click the image above for a video about arrays and loops.
## Arrays
Working with data is a common task for any language, and it's a much easier task when data is organized in a structural format, such as arrays. With arrays, data is stored in a structure similar to a list. One major benefit of arrays is that you can store different types of data in one array.
@ -191,6 +191,8 @@ Take a minute to watch a video on using `const`, `let` and `var`
[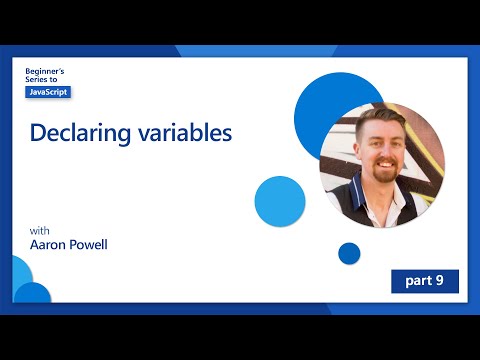](https://youtube.com/watch?v=JNIXfGiDWM8 "Types of variables")
> Click the image above for a video about variables.
### Add start logic
To begin the game, the player will click on start. Of course, we don't know when they're going to click start. This is where an [event listener](https://developer.mozilla.org/docs/Web/API/EventTarget/addEventListener) comes into play. An event listener will allow us to listen for something to occur (an event) and execute code in response. In our case, we want to execute code when the user clicks on start.
@ -151,6 +151,8 @@ Here's a quick video about `async`:
[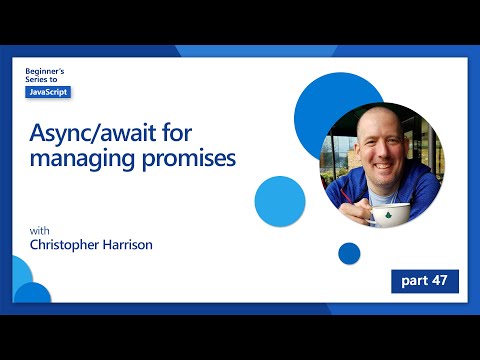](https://youtube.com/watch?v=YwmlRkrxvkk "Async and Await for managing promises")
> Click the image above for a video about async/await.
@ -181,6 +181,8 @@ Here's a quick video about `async/await` usage:
[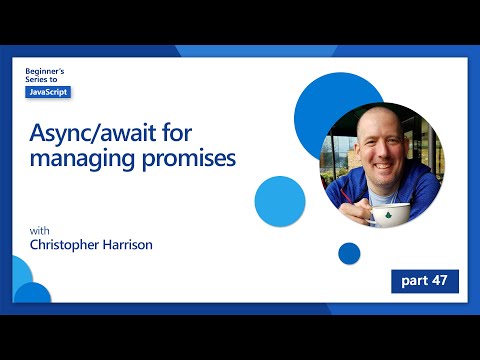](https://youtube.com/watch?v=YwmlRkrxvkk "Async and Await for managing promises")
> Click the image above for a video about async/await.
We use the `fetch()` API to send JSON data to the server. This method takes 2 parameters:
- The URL of the server, so we put back `//localhost:5000/api/accounts` here.