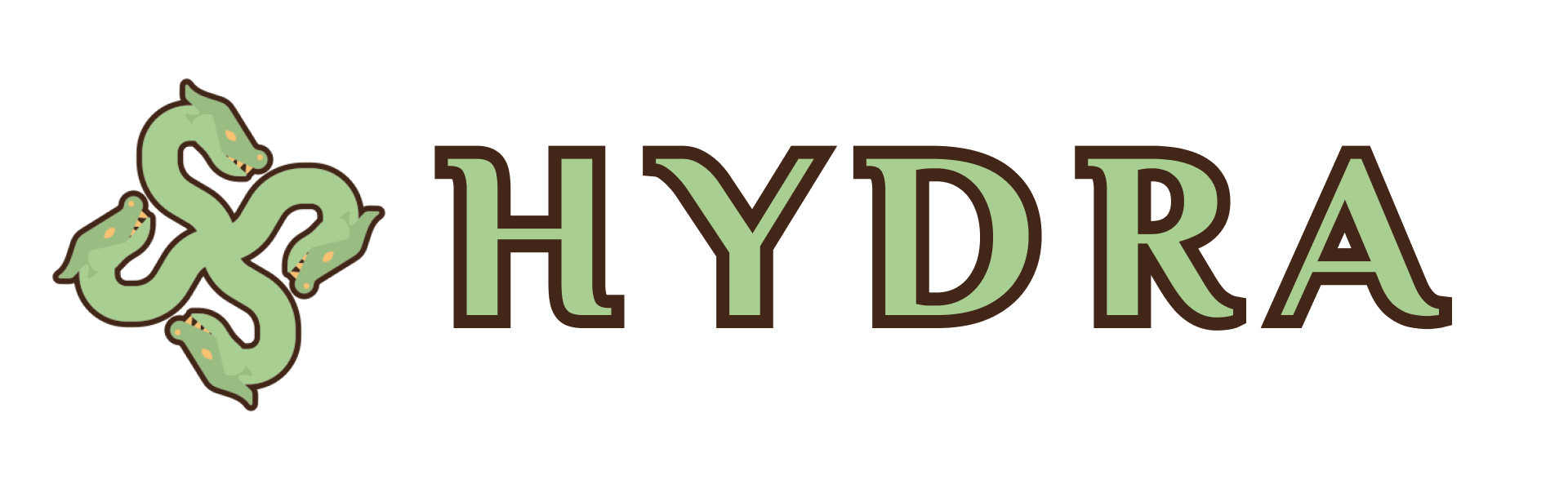
Hydra is a zero-config API boilerplate with Laravel Sanctum and comes with excellent user and role management API out of the box. Start your next big API project with Hydra, focus on building business logic, and save countless hours of writing boring user and role management API again and again.
Please note that the default admin user is **admin@hydra.project** and default password is **hydra**. You should create a new admin user before deploying to production and delete this default admin user. You can do that using available Hydra user management API, or using any DB management tool.
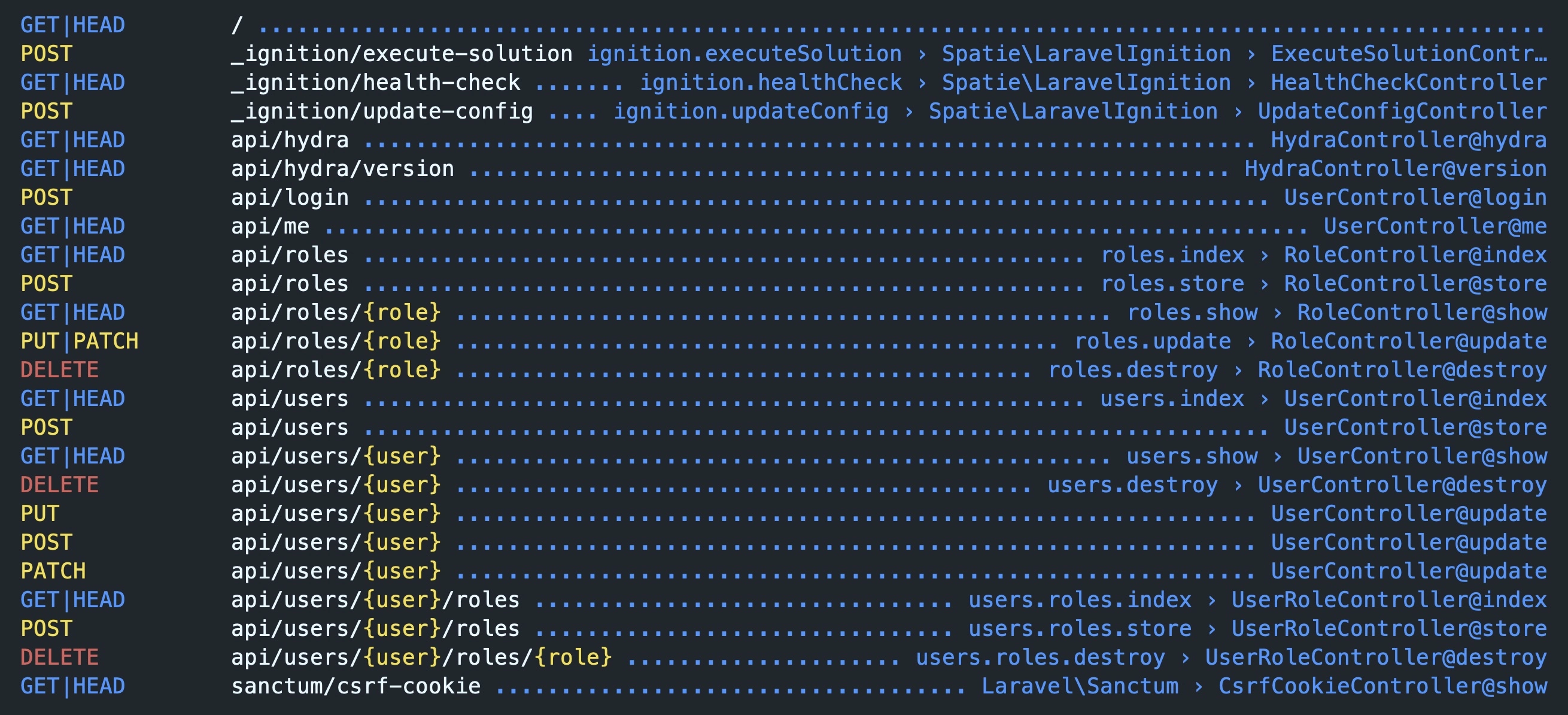
## Default Roles
Hydra comes with these `super-admin`,`admin`,`editor`,`customer` &`user` roles out of the box. For details, open the roles table after database seeding, or simply open laravel tinker and experiment with `Role` model
Let's have a look at what Hydra has to offer. Before experimenting with the following API endpoints, run your Hydra project using `php artisan serve` command. For the next part of this documentation, we assumed that Hydra is listening at http://localhost:8000
### User Registration
You can make an `HTTP POST` call to the following endpoint to create/register a new user. newly created user will have the `user` role by default.
```shell
http://localhost:8000/api/users
```
**API Payload & Response**
You can send a Form Multipart payload or a JSON payload like this
```json
{
"name":"Hydra User",
"email":"user@hydra.project",
"passsword":"Surprisingly A Good Password"
}
```
Voila! your user has been created and is now ready to login!
To list the roles, make an `HTTP GET` call to the following route, with Admin Token obtained from Admin Login. Add this token as a standard `Bearer Token` to your API call.
```shell
http://localhost:8000/api/roles
```
**API Payload & Response**
No payload required for this call.
You will get a JSON response with all the roles available in your project.
```json
[
{
"id": 1,
"name": "Administrator",
"slug": "admin"
},
{
"id": 2,
"name": "User",
"slug": "user"
},
{
"id": 3,
"name": "Customer",
"slug": "customer"
},
{
"id": 4,
"name": "Editor",
"slug": "editor"
},
{
"id": 5,
"name": "All",
"slug": "*"
},
{
"id": 6,
"name": "Super Admin",
"slug": "super-admin"
}
]
```
For any unsuccsesful attempt or wrong token, you will receive a 401 error response.
```json
{
"message": "Unauthenticated."
}
```
### Add a New Role (Admin Ability Required)
To list the roles, make an `HTTP POST` call to the following route, with Admin Token obtained from Admin Login. Add this token as a standard `Bearer Token` to your API call.
```shell
http://localhost:8000/api/roles
```
**API Payload & Response**
You need to supply title of the role as `name`, role `slug` in your payload as Multipart Form or JSON data
```json
{
"name":"Manager",
"slug":"manager"
}
```
For successful execution, you will get a JSON response with this newly created role.
```json
{
"name": "Manager",
"slug": "manager",
"id": 7
}
```
If this role `slug` already exists, you will get a 409 error message like this
```json
{
"error": 1,
"message": "role already exists"
}
```
For any unsuccsesful attempt or wrong token, you will receive a 401 error response.
```json
{
"message": "Unauthenticated."
}
```
### Update a Role (Admin Ability Required)
To list the roles, make an `HTTP PUT` or `HTTP PATCH` call to the following route, with Admin Token obtained from Admin Login. Add this token as a standard `Bearer Token` to your API call.
```shell
http://localhost:8000/api/roles/{roleid}
```
For example to update the Customer role, use this endpoint `http://localhost:8000/api/roles/3`
**API Payload & Response**
You need to supply title of the role as `name`, and/or role `slug` in your payload as Multipart Form or JSON data
```json
{
"name":"Product Customer",
"slug":"product-customer"
}
```
For successful execution, you will get a JSON response with this updated role.
```json
{
"id": 3,
"name": "Product Customer",
"slug": "product-customer"
}
```
Please note that you cannot change a `super-admin` or `admin` role slug because many API routes in Hydra exclusively require this role to function properly.
For any unsuccsesful attempt or wrong token, you will receive a 401 error response.