diff --git a/controller/kefu.go b/controller/kefu.go
index c6eefed..b974313 100644
--- a/controller/kefu.go
+++ b/controller/kefu.go
@@ -4,6 +4,7 @@ import (
"github.com/gin-gonic/gin"
"github.com/taoshihan1991/imaptool/models"
"github.com/taoshihan1991/imaptool/tools"
+ "strconv"
)
func GetKefuInfo(c *gin.Context){
@@ -34,9 +35,19 @@ func PostKefuInfo(c *gin.Context){
password:=c.PostForm("password")
avator:=c.PostForm("avator")
nickname:=c.PostForm("nickname")
+ roleId:=c.PostForm("role_id")
+ if roleId==""{
+ c.JSON(200, gin.H{
+ "code": 400,
+ "msg": "请选择角色!",
+ })
+ return
+ }
//插入新用户
if id==""{
- models.CreateUser(name,tools.Md5(password),avator,nickname)
+ uid:=models.CreateUser(name,tools.Md5(password),avator,nickname)
+ roleIdInt,_:=strconv.Atoi(roleId)
+ models.CreateUserRole(uid,uint(roleIdInt))
}else{
//更新用户
if password!=""{
diff --git a/models/user_roles.go b/models/user_roles.go
index 5ea3f53..f41e2ad 100644
--- a/models/user_roles.go
+++ b/models/user_roles.go
@@ -1,4 +1,9 @@
package models
+
+import (
+ "strconv"
+)
+
type User_role struct{
UserId string `json:"user_id"`
RoleId uint `json:"role_id"`
@@ -7,4 +12,11 @@ func FindRoleByUserId(userId interface{})User_role{
var uRole User_role
DB.Where("user_id = ?", userId).First(&uRole)
return uRole
+}
+func CreateUserRole(userId uint,roleId uint){
+ uRole:=&User_role{
+ UserId:strconv.Itoa(int(userId)),
+ RoleId: roleId,
+ }
+ DB.Create(uRole)
}
\ No newline at end of file
diff --git a/models/users.go b/models/users.go
index 1c6ab73..9af9d5f 100644
--- a/models/users.go
+++ b/models/users.go
@@ -11,14 +11,14 @@ type User struct {
Avator string `json:"avator"`
RoleName string `json:"role_name"`
}
-func CreateUser(name string,password string,avator string,nickname string){
+func CreateUser(name string,password string,avator string,nickname string)uint{
user:=&User{
Name:name,
Password: password,
Avator:avator,
Nickname: nickname,
}
- DB.Create(user)
+ return DB.Create(user).Value.(*User).ID
}
func UpdateUser(id string,name string,password string,avator string,nickname string){
user:=&User{
@@ -46,7 +46,7 @@ func DeleteUserById(id string){
}
func FindUsers()[]User{
var users []User
- DB.Order("id desc").Find(&users)
+ DB.Select("user.*,role.name role_name").Joins("join user_role on user.id=user_role.user_id").Joins("join role on user_role.role_id=role.id").Order("user.id desc").Find(&users)
return users
}
func FindUserRole(query interface{},id interface{})User{
diff --git a/readme.md b/readme.md
index b0349b1..dde8dba 100644
--- a/readme.md
+++ b/readme.md
@@ -27,6 +27,8 @@
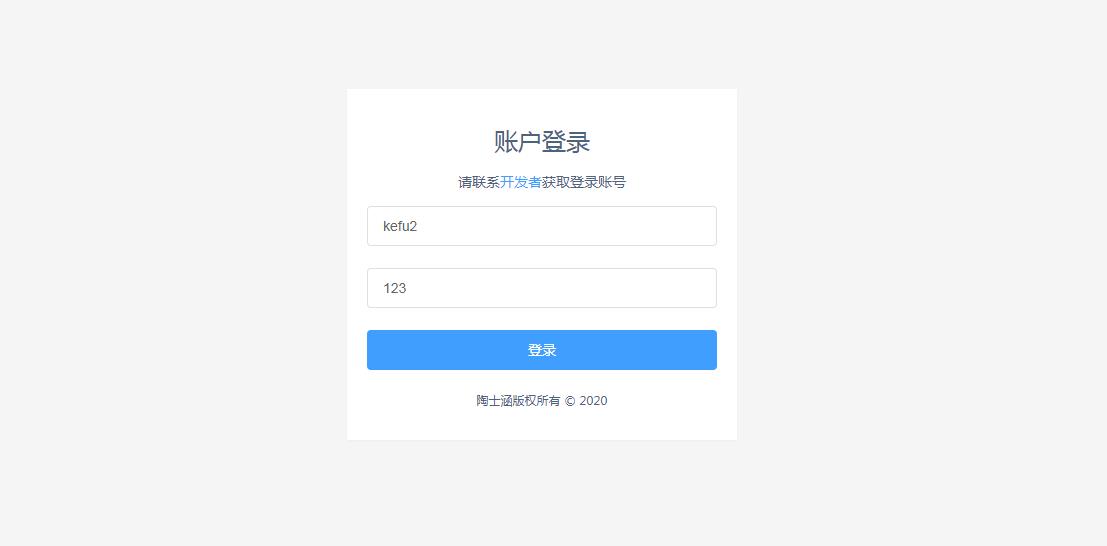
+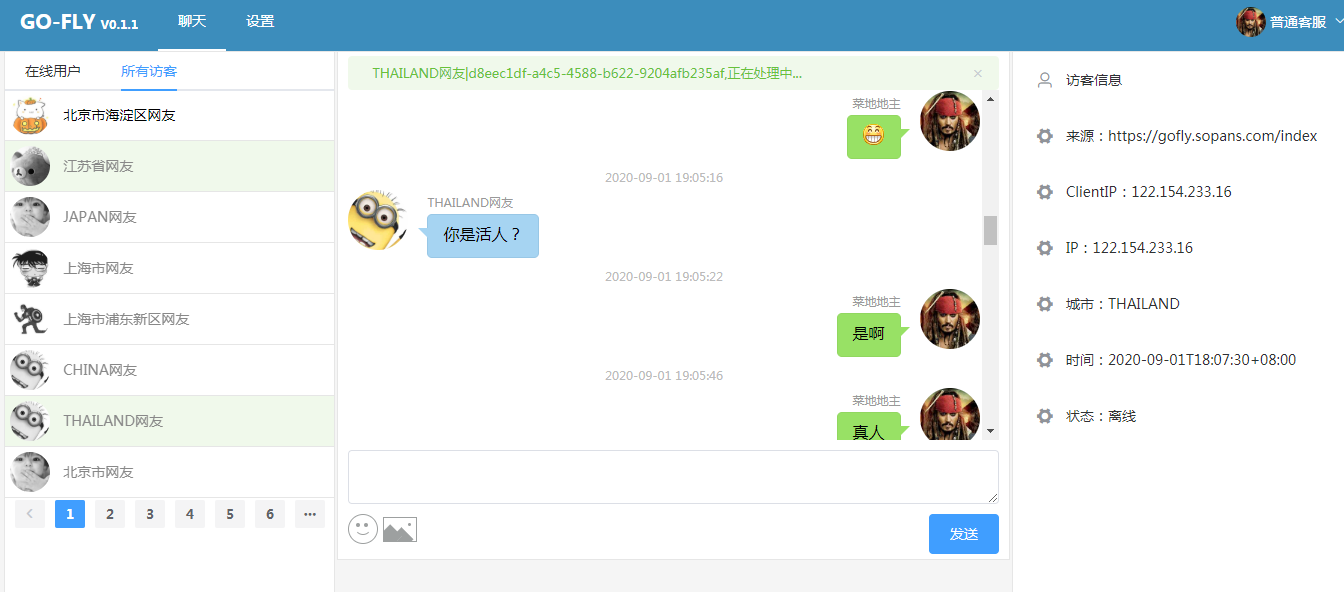
+
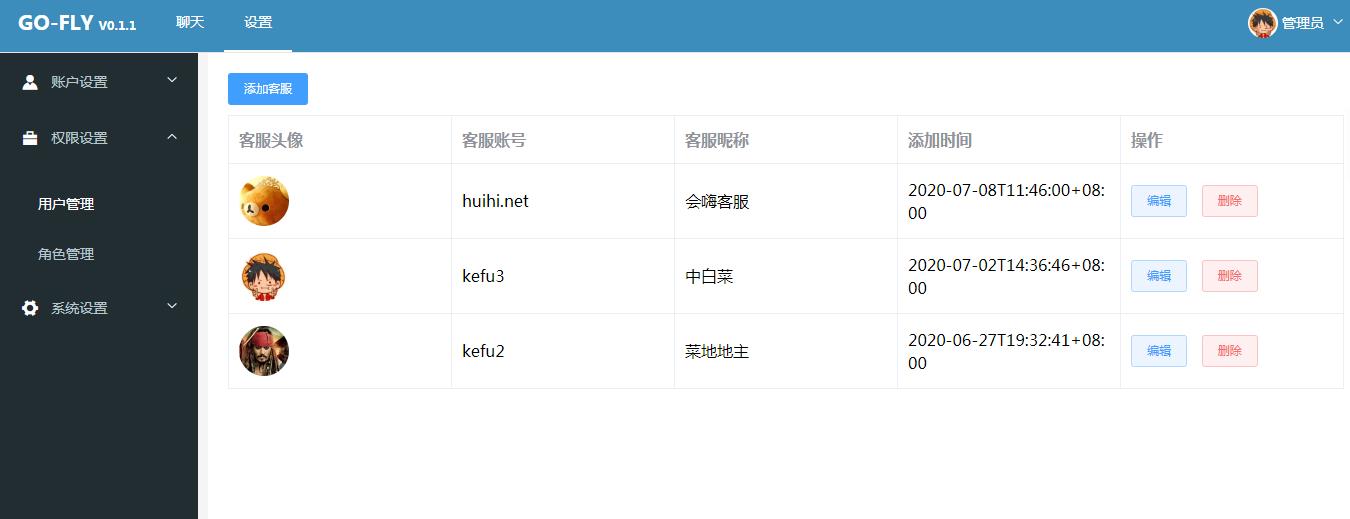
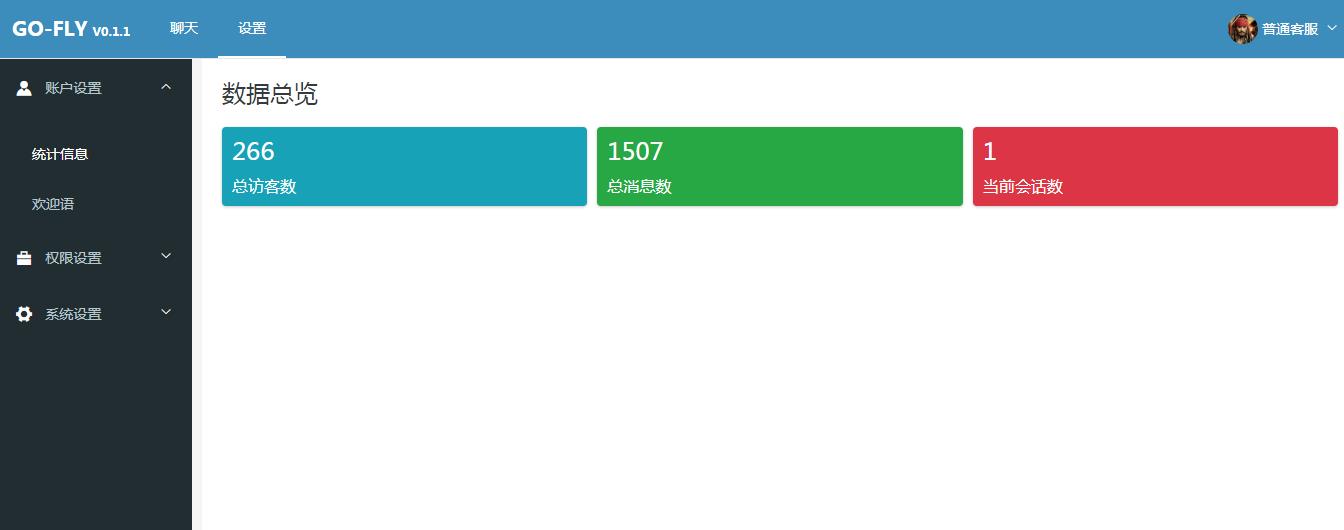
diff --git a/static/html/setting_bottom.html b/static/html/setting_bottom.html
index dd8bdca..6369127 100644
--- a/static/html/setting_bottom.html
+++ b/static/html/setting_bottom.html
@@ -60,10 +60,15 @@
password:"",
avator:"",
nickname:"",
+ role_name:"",
},
roleList:[],
roleDialog:false,
noticeList:[],
+ welcomeDialog:false,
+ welcomeForm: {
+ content: "",
+ },
roleForm:{
id:"",
name:"",
@@ -146,6 +151,10 @@
showClose:true,
});
},
+ addWelcome(){
+ this.welcomeForm.content="";
+ this.welcomeDialog=true;
+ },
//初始化数据
initInfo(){
let _this=this;
@@ -162,6 +171,9 @@
this.sendAjax("/kefulist","get",{},function(result){
_this.kefuList=result;
});
+ this.sendAjax("/roles","get",{},function(result){
+ _this.roleList=result;
+ });
}
if(ACTION=="roles_list"){
this.sendAjax("/roles","get",{},function(result){
diff --git a/static/html/setting_kefu_list.html b/static/html/setting_kefu_list.html
index 2bbdc9e..d8b7447 100644
--- a/static/html/setting_kefu_list.html
+++ b/static/html/setting_kefu_list.html
@@ -25,6 +25,10 @@
prop="nickname"
label="客服昵称">
+
+
@@ -63,6 +67,12 @@
+
+
+
+
+
+