[<< Day 11](../11_Day_Events/11_events.md) | [Day 13 >>](../13_Day_Controlled_Versus_Uncontrolled_Input/13_uncontrolled_input.md)
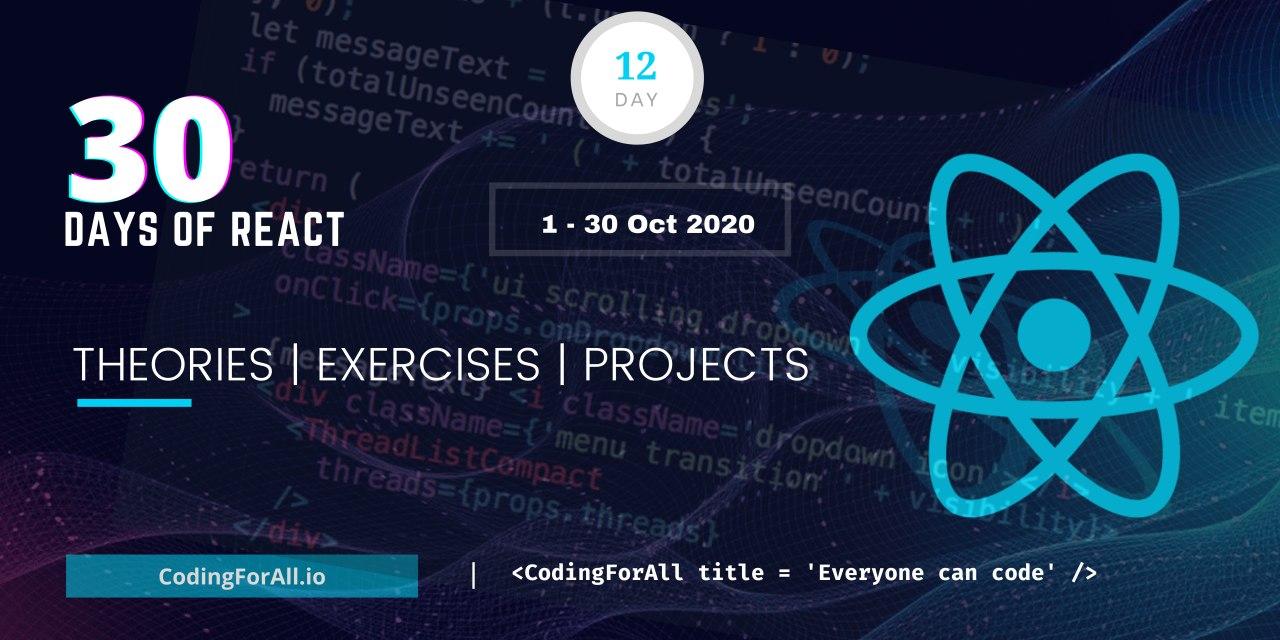
- [Forms](#forms)
- [Getting data from an input field](#getting-data-from-an-input-field)
- [Getting multiple input data from form](#getting-multiple-input-data-from-form)
- [Get data from different input field types](#get-data-from-different-input-field-types)
- [Form Validation](#form-validation)
- [What is validation?](#what-is-validation)
- [What is the purpose of validation](#what-is-the-purpose-of-validation)
- [Validation Types](#validation-types)
- [Exercises](#exercises)
- [Exercises: Level 1](#exercises-level-1)
- [Exercises: Level 2](#exercises-level-2)
- [Exercises: Level 3](#exercises-level-3)
# Forms
Form is used to collect data from a user. Once in a while we use form to fill our information on a paper or on a website. Either to sign up, sign in or to apply for a job we fill different form fields to submit our data to remote database. We encounter different form fields when we fill a form such as simple text, email, password, telephone, date, checkbox, radio button, option selection and text area field. Currently, HTML5 has provide quite a lot of field types. You may have a look at the following available HTML5 input types.
```html
```
Another HTML fields to get data from a form are textarea and select with options elements.
```html
```
Now, you know most of the fields we need to get data from a form. Let's start with an input with type text field. In the previous day, we saw different types of events and today we will focus on more of _onChange_ event type which triggers whenever an input field data changes. Input field has by default a memory to store input data but in this section we control that using state and we implement a controlled input. Today we will implement a controlled input. We will cover uncontrolled input in a separate section.
## Getting data from an input field
So far we did not get any data from input field. Now, it is time to learn how to get data from an input field. We need an input field, event listener (onChange) and state to get data from a controlled input. See the example below. The h1 element below the input tag display what we write on the input. Check live [demo](https://codepen.io/Asabeneh/full/OJVpyqm).
The input element has many attributes such as value, name, id, placeholder, type and event handler. In addition, we can link a label and an input field using an id of input field and htmlFor of the label.If label and input are linked it will focus the input when we click on label. Look at the example give below.
```js
import React, { Component } from 'react'
import ReactDOM from 'react-dom'
class App extends Component {
// declaring state
// initial state
state = {
firstName: '',
}
handleChange = (e) => {
const value = e.target.value
this.setState({ firstName: value })
}
render() {
/*
accessing the state value and
this value will injected to the input in the value attribute
*/
const firstName = this.state.firstName
return (
{this.state.firstName}
)
}
}
const rootElement = document.getElementById('root')
ReactDOM.render(, rootElement)
```
We usually use form to handle user information. Let us move to form section and make use the form element.
## Getting multiple input data from form
In this section we will develop a small form which collect user information. Our user is a student. We use a parent form element and certain number of input elements to collect user information. In addition to that we will have event listener for the form (onSubmit) and for the inputs (onChange). See the following example try to see the commonts too. You can also check the live [demo](https://codepen.io/Asabeneh/full/eYNvJda).
```js
import React, { Component } from 'react'
import ReactDOM from 'react-dom'
class App extends Component {
// declaring initial state
state = {
firstName: '',
lastName: '',
country: '',
title: '',
}
handleChange = (e) => {
/*
we can get the name and value like this: e.target.name, e.target.value
but we can also destructure name and value from e.target
const name = e.target.name
const value = e.target.value
*/
const { name, value } = e.target
// [variablename] to use a variable name as a key in an object
// name refers to the name attribute of the input elements
this.setState({ [name]: value })
}
handleSubmit = (e) => {
/*
e.preventDefault()
stops the default behavior of form element
specifically refreshing of page
*/
e.preventDefault()
/*
the is the place where we connect backend api
to send the data to the database
*/
console.log(this.state)
}
render() {
// accessing the state value by destrutcturing the state
const { firstName, lastName, title, country } = this.state
return (
Add Student
)
}
}
const rootElement = document.getElementById('root')
ReactDOM.render(, rootElement)
```
The above form handles only text types but do have different input field types. Let's do another form which handle all the different input field types.
## Get data from different input field types
```js
// index.js
import React, { Component } from 'react'
import ReactDOM from 'react-dom'
const options = [
{
value: '',
label: '-- Select Country--',
},
{
value: 'Finland',
label: 'Finland',
},
{
value: 'Sweden',
label: 'Sweden',
},
{
value: 'Norway',
label: 'Norway',
},
{
value: 'Denmark',
label: 'Denmark',
},
]
// mapping the options to list(array) of JSX options
const selectOptions = options.map(({ value, label }) => (
))
class App extends React.Component {
// declaring state
state = {
firstName: '',
lastName: '',
email: '',
country: '',
tel: '',
dateOfBirth: '',
favoriteColor: '',
weight: '',
gender: '',
file: '',
bio: '',
skills: {
html: false,
css: false,
javascript: false,
},
}
handleChange = (e) => {
/*
we can get the name and value like: e.target.name, e.target.value
Wwe can also destructure name and value from e.target
const name = e.target.name
const value = e.target.value
*/
const { name, value, type, checked } = e.target
/*
[variablename] we can make a value stored in a certain variable could be a key for an object, in this case a key for the state
*/
if (type === 'checkbox') {
this.setState({
skills: { ...this.state.skills, [name]: checked },
})
} else if (type === 'file') {
console.log(type, 'cehck here')
this.setState({ [name]: e.target.files[0] })
} else {
this.setState({ [name]: value })
}
}
handleSubmit = (e) => {
/*
e.preventDefault()
stops the default behavior of form element
specifically refreshing of page
*/
e.preventDefault()
const {
firstName,
lastName,
email,
tel,
dateOfBirth,
favoriteColor,
weight,
country,
gender,
bio,
file,
skills,
} = this.state
const formattedSkills = []
for (const key in skills) {
console.log(key)
if (skills[key]) {
formattedSkills.push(key.toUpperCase())
}
}
const data = {
firstName,
lastName,
email,
tel,
dateOfBirth,
favoriteColor,
weight,
country,
gender,
bio,
file,
skills: formattedSkills,
}
/*
the is the place where we connect backend api
to send the data to the database
*/
console.log(data)
}
render() {
// accessing the state value by destrutcturing the state
const {
firstName,
lastName,
email,
tel,
dateOfBirth,
favoriteColor,
weight,
country,
gender,
bio,
} = this.state
return (
Add Student
)
}
}
const rootElement = document.getElementById('root')
ReactDOM.render(, rootElement)
```
## Form Validation
## What is validation?
The action or process of checking or proving the validity or accuracy of something in this case data.
## What is the purpose of validation
The main purpose to validation is to get a desired data from users. In addition, to prevent malicious users and data.
## Validation Types
Validation can be done in client side or sever side. At the moment, we are using React which is a front end technology and we use client side validation.A validation can implement using HTML5 built-in validation or using JavaScript(using regular expression).
In the following snippet of code, a validation has been implemented the first field. Try to understand how it works. The onBlur event has been used to check validity when the input is not focused.
```js
// index.js
import React, { Component } from 'react'
import ReactDOM from 'react-dom'
const options = [
{
value: '',
label: '-- Select Country--',
},
{
value: 'Finland',
label: 'Finland',
},
{
value: 'Sweden',
label: 'Sweden',
},
{
value: 'Norway',
label: 'Norway',
},
{
value: 'Denmark',
label: 'Denmark',
},
]
// mapping the options to list(array) of JSX options
const selectOptions = options.map(({ value, label }) => (
))
class App extends Component {
// declaring state
state = {
firstName: '',
lastName: '',
email: '',
country: '',
tel: '',
dateOfBirth: '',
favoriteColor: '',
weight: '',
gender: '',
file: '',
bio: '',
skills: {
html: false,
css: false,
javascript: false,
},
touched: {
firstName: false,
lastName: false,
},
}
handleChange = (e) => {
/*
we can get the name and value like: e.target.name, e.target.value
Wwe can also destructure name and value from e.target
const name = e.target.name
const value = e.target.value
*/
const { name, value, type, checked } = e.target
/*
[variablename] we can make a value stored in a certain variable could be a key for an object, in this case a key for the state
*/
if (type === 'checkbox') {
this.setState({
skills: { ...this.state.skills, [name]: checked },
})
} else if (type === 'file') {
this.setState({ [name]: e.target.files[0] })
} else {
this.setState({ [name]: value })
}
}
handleBlur = (e) => {
const { name, value } = e.target
this.setState({ touched: { ...this.state.touched, [name]: true } })
}
validate = () => {
// Object to collect error feedback and to display on the form
const errors = {
firstName: '',
}
if (
(this.state.touched.firstName && this.state.firstName.length < 3) ||
(this.state.touched.firstName && this.state.firstName.length > 12)
) {
errors.firstName = 'First name must be between 2 and 12'
}
return errors
}
handleSubmit = (e) => {
/*
e.preventDefault()
stops the default behavior of form element
specifically refreshing of page
*/
e.preventDefault()
const {
firstName,
lastName,
email,
country,
gender,
tel,
dateOfBirth,
favoriteColor,
weight,
bio,
file,
skills,
} = this.state
const formattedSkills = []
for (const key in skills) {
console.log(key)
if (skills[key]) {
formattedSkills.push(key.toUpperCase())
}
}
const data = {
firstName,
lastName,
email,
country,
gender,
tel,
dateOfBirth,
favoriteColor,
weight,
bio,
file,
skills: formattedSkills,
}
/*
the is the place where we connect backend api
to send the data to the database
*/
console.log(data)
}
render() {
// accessing the state value by destrutcturing the state
// the noValidate attribute on the form is to stop the HTML5 built-in validation
const { firstName } = this.validate()
return (
Add Student
)
}
}
const rootElement = document.getElementById('root')
ReactDOM.render(, rootElement)
```
# Exercises
## Exercises: Level 1
1. What is the importance of form?
2. How many input types do you know?
3. Mention at least four attributes of an input element
4. What is the importance of htmlFor?
5. Write an input type which is not given in the example if there is?
6. What is a controlled input?
7. What do you need to write a controlled input?
8. What event type do you use to listen changes on an input field?
9. What is the value of a checked checkbox?
10. When do you use onChange, onBlur, onSubmit?
11. What is the purpose of writing e.preventDefault() inside the submit handler method?
12. How do you bind data in React? The first input field example is data binding in React.
13. What is validation?
14. What is the event type you use to listen when an input changes?
15. What are event types do you use to validate an input?
## Exercises: Level 2
1. Validate the form given above (a gif image or a video will be provided later). First try to validate without using any library then try it with [validator.js](https://www.npmjs.com/package/validator).
## Exercises: Level 3
Coming ..
🎉 CONGRATULATIONS ! 🎉
[<< Day 11](../11_Day_Events/11_events.md) | [Day 13 >>](../13_Day_Controlled_Versus_Uncontrolled_Input/13_uncontrolled_input.md)